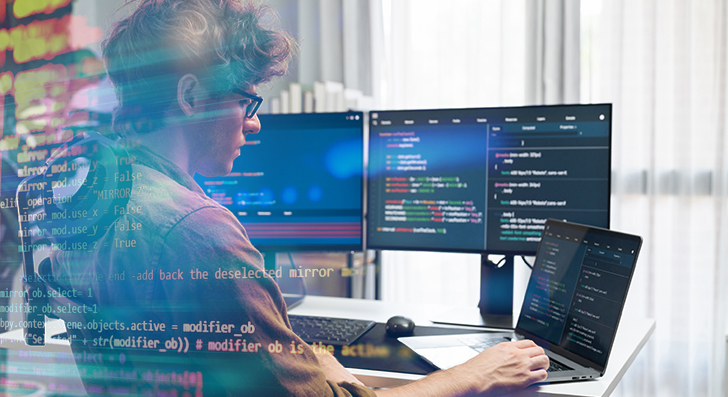
Scalability indicates your application can manage development—more buyers, far more info, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and sensible guideline that may help you commence by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be portion of your prepare from the beginning. A lot of applications fall short when they increase fast mainly because the initial design can’t take care of the additional load. Like a developer, you might want to Believe early regarding how your system will behave under pressure.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As an alternative, use modular style and design or microservices. These patterns split your application into smaller, impartial sections. Each module or support can scale By itself without the need of affecting The entire system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 buyers or just a hundred? Pick the right sort—relational or NoSQL—based upon how your details will develop. Program for sharding, indexing, and backups early, Even though you don’t have to have them yet.
A different important stage is in order to avoid hardcoding assumptions. Don’t generate code that only functions below latest conditions. Think of what would come about In case your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that support scaling, like message queues or event-driven techniques. These support your app manage a lot more requests devoid of finding overloaded.
Any time you Create with scalability in mind, you're not just planning for achievement—you are lowering long term headaches. A perfectly-prepared procedure is simpler to keep up, adapt, and mature. It’s better to arrange early than to rebuild afterwards.
Use the best Database
Choosing the right databases is often a essential Component of constructing scalable programs. Not all databases are built the same, and utilizing the Improper you can sluggish you down and even cause failures as your application grows.
Start out by knowing your data. Can it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically solid with associations, transactions, and regularity. They also aid scaling strategies like read replicas, indexing, and partitioning to manage more website traffic and information.
If the facts is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured info and will scale horizontally more simply.
Also, consider your go through and generate patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can deal with substantial generate throughput, or even occasion-dependent information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe forward. You may not need to have Highly developed scaling features now, but choosing a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And normally observe databases general performance as you grow.
In short, the right databases relies on your application’s composition, pace demands, And exactly how you hope it to mature. Acquire time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Speedy code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most intricate Answer if a straightforward one particular will work. Maintain your functions small, targeted, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular across substantial tables.
In the event you observe a similar info staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with large datasets. Code and queries that perform wonderful with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout various servers. In lieu of just one server executing every one of the operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical info all over again—like a product page or maybe a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter generally. And usually make sure your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your application manage additional users, remain rapid, and Get better from issues. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term more info capability. When targeted traffic boosts, you may increase extra assets with just a couple clicks or routinely employing car-scaling. When targeted traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are another vital Instrument. A container offers your application and anything it should run—code, libraries, settings—into a person device. This makes it easy to maneuver your application in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They conserve time, lower risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t keep track of your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better conclusions as your application grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it's going to take for users to load pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Set up alerts for essential issues. For instance, In case your response time goes higher than a Restrict or maybe a assistance goes down, it is best to get notified quickly. This will help you resolve difficulties rapidly, usually just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it leads to serious problems.
As your app grows, traffic and facts boost. With out checking, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications will need a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you may Develop apps that mature easily devoid of breaking stressed. Commence smaller, think massive, and Establish intelligent.